C# Array
C# array is a kind of variable that stores a list of addresses of variables and can store a set of data having the same data type. Declaring multiple variables with the same type and used for the same purpose can be exhausting. What if you want to store a thousand values with integers and they will be used, for example, a list of grades of students. It would be tedious to declare all of those one thousand variables. But with C# Array, you can declare them in one line. Below shows you the simplest way to declare a C# Array.
datatype[] arrayName = new datatype[length];
The datatype is the type of variable that the array will store. The square brackets next to the data type tell that it is a C# Array. The arrayName is the identifier or the name of the variable. When naming a C# Array, it is a good practice to make it plural to indicate that it is a C# Array. For example, use numbers instead of number when naming a C# Array. The length tells the compiler how many data or values you are going to store in an array. We used the new keyword to allocate enough spaces for the C# Array in the memory depending on the value specified by the length. Each item in an array is called an array element. If we are to declare an array that can store 5 values of type integer, we would write:
int[] numbers = new int[5];
This declaration reserves 5 addresses inside your computer’s memory waiting for a value to be stored. So how can we store values to each of the array elements? Array’s value can be accessed and modified by indicating their index or position.
numbers[0] = 1;
numbers[1] = 2;
numbers[2] = 3;
numbers[3] = 4;
numbers[4] = 5;
Index starts with 0 and ends with (length – 1) of the array. For example, we have 5 elements so the indices of our C# Array start from 0 and ends with 4 because length – 1 equates to 5 – 1 which is 4. That means that index 0 is the first element, index 1 is the second element and so on. Look at the image below to better understand this concept.
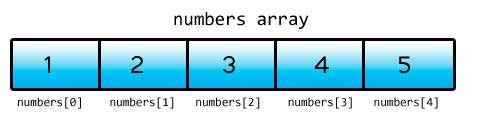
Consider each square is an array element and the values inside them are the values they contain. Newbie programmers often get confused by the index resulting to errors such as starting the count from 1 to 5. If you access an array element with an index that’s not inside the range of valid indexes, then you will get an IndexOutOfRangeException which simply says that you are accessing an address that does not exist. Just remember the formula and you won’t have any problems.
If you want to assign values immediately in the declaration of a variable, you can do a modified version of the declaration of an array.
datatype[] arrayName = new datatype[length] { val1, val2, ... valN };
You can enumerate the values inside curly braces immediately after the declaration of the size of the array. You should separate the values with commas. The number of values inside the curly braces should exactly match the size of the array declared. Let’s look at an example.
int[] numbers = new int[5] { 1, 2, 3, 4, 5 };
This will have the same results as the code in Figure 1 but it save us a lot of lines of code. You can still change the values of any element by accessing its index and assigning a new value. Our numbers C# Array requires 5 elements so we supplied it with 5 values. If we are to supply less or more than it requires, we will encounter an error. There is another way of declaring an array. You can supply an array with any number of values as long as you do not indicate the size. Here’s an example:
int[] numbers = new int[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
We are now giving 10 values to the C# Array. Notice that there’s no length indicated for the array. If this is the case, the compiler will count the number of values inside the curly braces and that will determine the length of the array. Note that if you do not give a length for the array, you need to supply values for it. If not, you will receive an error.
int[] numbers = new int[]; //not allowed
There is a shorter version for doing this:
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
We simply assign the values inside curly braces without using the new keyword and the data type. This will also assign all of the 10 values into the array and automatically counts the length that the array should have.
Accessing C# Array Values Using for Loop
The following is an example of using arrays. Our program will retrieve 5 values from the user and then calculate the average of those numbers.
using System;
namespace ArraysDemo
{
public class Program
{
public static void Main()
{
int[] numbersone = new int[5];
int total = 0;
double average;
for (int i = 0; i < numbersone.Length; i++)
{
Console.Write("Enter a number: ");
numbers[i] = Convert.ToInt32(Console.ReadLine());
}
for (int i = 0; i < numbersone.Length; i++)
{
total += numbersone[i];
}
average = total / (double)numbersone.Length;
Console.WriteLine("Average = {0}", average);
}
}
}
Example 1 – Using C# Array
Enter a number: 90 Enter a number: 85 Enter a number: 80 Enter a number: 87 Enter a number: 92 Average = 86
Line 9 declares an array that can hold 5 integer values. Lines 10 and 11 declares variables that will be used on calculating the average. Note that total is initialized to 0 to avoid errors when adding values to it. Lines 13-17 uses a for loop that will repeat on getting input from the user. We used the Length property of the array to determine the number of elements it contains. Although we can simply specify the value 5 in the condition, using the Length property is more versatile as we can change the length of the array and the condition in the for loop will adjust to the new change. Line 16 retrieves input from the user, converts it to int, and stores it on an array element. The index uses the current value of i. For example, at the beginning of the loop, i is initialized to 0, so when Line 16 is first encountered, it will add the value retrieve by the user to numbers[0]. After the loop, i is incremented by 1, and one Line 16 is reached again, it will now assign the retrieved value to numbers[1]. It will continue as long as the for loop meets the condition.
Lines 19-22 uses another for loop to access each value of the numbers array. We used the same technique of using the counter variable as the index. Each element of the numbers is added to the variable total. After the end of the loop, we can now calculate the average (line 24). We divide the value of total by the number of elements of the numbers array. To access the number of elements of numbers, we used again the Length property of the array. Note that we converted the value of the Length property to double so the expression will yield a double result which includes the fractional part of the result. If we don’t convert any of the operands of the division, then it will yield an integer result which does not include the fractional or decimal part of the result. Line 26 displays the calculated average.
Array’s length cannot be changed once it was initialized. For example, if you initialize an array to contain only 5 elements, then you cannot resize the array to contain, for example, 10 elements. A special set of classes acts the same as the array but is capable of resizing the number of elements they can contain. Arrays are very useful in many situations and it is important that you master this concept and how they can be used.