FolderBrowserDialog Control
The Folder Browser Dialog control (System.Windows.Forms.FolderBrowserDialog) allows you to browse for a directory in your system. The control uses a tree view to show all the folders. The Browser shows the Desktop (by default) as the top level root folder and below it is its content. You can click the arrows or each folder to show other folders/subdirectories inside it. You can also create a new folder inside the selected folder or directory.
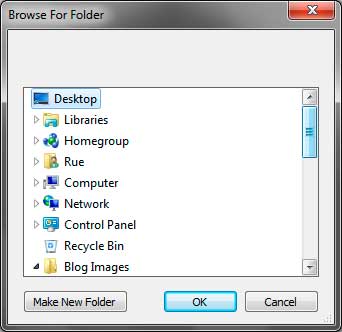
The following are some of the useful properties of the Folder Browser Dialog control.
Property | Description |
---|---|
Description | Allows you to add a descriptive text above the tree view of the Folder Browser Dialog. |
RootFolder | Gets or sets the folder that the Folder Browser Dialog will consider as the root or the top level folder. |
SelectedPath | The folder or path that the user has selected. |
ShowNewFolderButton | Shows or hides the Make New Folder button. |
The Description property allows you to add a description right above the tree view of the dialog.
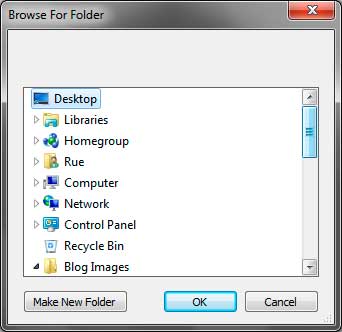
If you don’t want the desktop as the top level root folder, then you can use the RootFolder property. In the properties window, find the RootFolder property and press the drop down button beside it. You will be shown with predefined paths that you can choose.
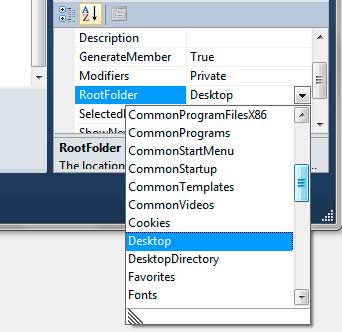
You can retrieve the selected folder or path using the SelectedPath property. The path is returned as a string.
We will now create an example application to show the use Folder Browser Dialog control. Our example program will allow the user to choose a directory, then it will be displayed in a text box. The contents of the directory will then be listed using a list box. Create a new form similar to the one below. which contains a button, text box, and a list box control.
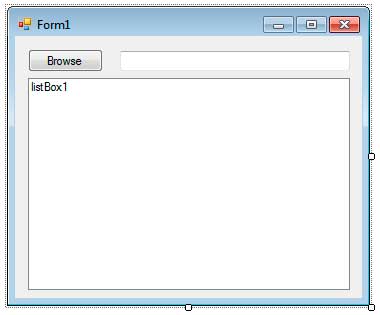
Add the FolderBrowserDialog from the Dialogs category of the Toolbox. The control will be located below the bottom portion of the Designer. Double click the Browse button and use the code below for the event handler.
private void button1_Click(object sender, EventArgs e)
{
DialogResult result = folderBrowserDialog1.ShowDialog();
if (result == DialogResult.OK)
{
//Show the path using the text box
textBox1.Text = folderBrowserDialog1.SelectedPath;
//Obtain information about the path
DirectoryInfo selectedPath = new DirectoryInfo(textBox1.Text);
//Clear the list box first
listBox1.Items.Clear();
//Check if there are directories then add a label
if (selectedPath.GetDirectories().Length > 0)
listBox1.Items.Add("== Directories ==");
//Show all the directories using the ListBox control
foreach (DirectoryInfo dir in selectedPath.GetDirectories())
{
//Show only the name of the directory
listBox1.Items.Add(dir.Name);
}
//Check if there are files then add a label
if (selectedPath.GetFiles().Length > 0)
listBox1.Items.Add("== Files ==");
//Show all the directories using the ListBox control
foreach (FileInfo file in selectedPath.GetFiles())
{
listBox1.Items.Add(file.Name);
}
}
}
Please note that we also need to import the System.IO namespace above the code.
using System.IO;
First, we called the FolderBrowserDialog using the ShowDialog method. The user can now pick a folder and press OK. We need to test if the user pressed the OK button. This can be determined using the value returned by the ShowDialog method. If the user presses the OK button, we display the selected path in the text box.
DirectoryInfo selectedPath = new DirectoryInfo(textBox1.Text);
This line creates a new DirectoryInfo object using the path selected by the user. A DirectoryInfo object is contained in the System.IO namespace. It contains properties that describes a directory such as the full path of the directory and its contents. We clear the contents of the list box in case there are some previously added items exists.
//Check if there are directories then add a label
if (selectedPath.GetDirectories().Length > 0)
listBox1.Items.Add("== Directories ==");
//Show all the directories using the ListBox control
foreach (DirectoryInfo dir in selectedPath.GetDirectories())
{
//Show only the name of the directory
listBox1.Items.Add(dir.Name);
}
We then check if there are subdirectories inside the current folder using the Length property of the DirectoryInfo[] value returned by calling the GetDirectories method. If so, we create a label and add it to our list box. We enumerate all the subdirectories inside the current folder using the GetDirectories method and a foreach loop. loop. We add the name of each subdirectory to the list box.
//Check if there are files then add a label
if (selectedPath.GetFiles().Length > 0)
listBox1.Items.Add("== Files ==");
//Show all the directories using the ListBox control
foreach (FileInfo file in selectedPath.GetFiles())
{
listBox1.Items.Add(file.Name);
}
We used the same technique for enlisting all the files inside the selected folder. But this time, we use the GetFiles method and FileInfo objects. The FileInfo object contains information about a specified file.
Now execute the program and select a folder. Press OK and look as the program shows you the directories and folders contained inside the selected folder.