Keyboard Events
When you want to handle events when pressing keys in the keyboard, you can handle the KeyPress, KeyDown, and KeyUp events. The KeyDown event occurs when a keyboard key is pressed down and the KeyUp event occurs after you release the pressed key. The KeyPress event triggers when a complete keypress is made(pressing then releasing the key). The following example adds a KeyPressevent to the form and whenever a key is pressed in the keyboard, it is added to the text of a label. Create a new Windows Forms Application and name it KeyBoardEvents then add a Label control.
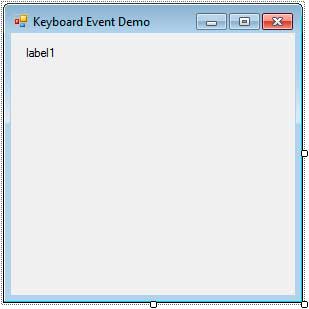
Remove the text of the Label. Select the form and in the Properties Window, find the KeyPress event and double click it to create an event handler. Use the code inside the Form1_KeyPress event handler of the code below.
using System;
using System.Windows.Forms;
namespace KeyBoardEvents
{
public partial class Form1 : Form
{
//Variable to count letters for simple word wrap
private int charCount = 0;
public Form1()
{
InitializeComponent();
}
private void Form1_KeyPress(object sender, KeyPressEventArgs e)
{
charCount++;
//Go to next line after the line's 30th character
if (charCount > 30)
{
label1.Text += "\r\n";
charCount = 0;
}
else
{
//Append the pressed keyboard key to the label using KeyChar property
label1.Text += e.KeyChar;
}
}
}
}
The KeyPress event will trigger whenever a button in your keyboard is pressed. Line 9 declares and initialize a counter variable named charCount that will be used to detect the number of characters of the current line This will be used for our simple word wrapping mechanism. The event handler monitors the number of characters typed by incrementing the charCount at Line 18. The condition at Line 21 tests whether the value of charCount doesn’t exceed 30. If so, it goes to the next line by adding the “\r\n” (carriage return-line feed) which is Windows’ way of proceeding to the next line. We then reset the charCount to 0 (Line 24) since we reached the beginning of the next line. If the charCount is still below or equal to 30, then we simply append the character typed by the user by using the KeyPressEventArgs.KeyChar property. When you execute the program, you can type letters using your keyboard and watch as the text inside the label is updated and the key you have just entered was appended to its text.
When you handle the KeyDown and KeyUp events, you get a different event argument named KeyEventArgs and it contains more properties about the pressed key. It contains the following properties.
Property | Description |
---|---|
Alt | Determines if the Alt button is pressed. |
Control | Determines if the Control button is pressed. |
KeyCode | Gets the Keys value of the key that was pressed. It is used to detect a specific key that is pressed. |
KeyData | Similar to the KeyCode property but also records the modifier flags (SHIFT, CTRL, ALT) that are pressed. |
KeyValue | Returns the numeric representation of the key that was pressed. |
Modifier | Determines which combination of modifiers flags (SHIFT, CTRL, ALT) is pressed. |
Shift | Tells whether the Shift key is pressed. |
SuppressKeyPress | Allows you to prevent the user from giving an input from the keyboard. |
As an example, the following code snippet uses the SupressKeyPress property of the KeyEventArgs to only allow numeric input and disallow any other characters such as alphabet or symbols. Add a text box to your form and add an event handler to its KeyDown event.
private void textBox1_KeyDown(object sender, KeyEventArgs e)
{
if (!(e.KeyCode >= Keys.D0 && e.KeyCode <= Keys.D9 && !e.Shift))
{
e.SuppressKeyPress = true;
}
}
The condition inside the if statement says that if the key typed by the user is not a number key, or if the shift key is pressed, then it will be ignored by setting the SuppressKeyPress property. We used the KeyCode property in the condition which contains values from the Keys enumeration. The numeric keys are represented by values D0 to D9. The need to check if the Shift key is not pressed is needed since pressing Shift key and a numeric key will actually result in a symbol associated to the number key (@ to 2 for example). Therefore, we used the Shift property of the KeyEventArgs class.