ListBox Control
The ListBox control is used to show a list of strings which you can select. By default, you can only select one item. The List Box control is best used if you are trying to display a large number of items. The following are the commonly used properties of the ListBox control.
Property | Description |
---|---|
ColumnWidth | Specifies the width of each column if MultiColumn is set to true. |
DataSource | Specifies the source of data that the List Box will display. |
Items | Contains the items that the List Box will display. |
MultiColumn | Tells whether the List Box supports multiple columns. |
SelectedIndex | The zero-based index of the selected item. |
SelectedIndices | Contains the zero-based index of each selected item. |
SelectedItem | Returns the selected item as an object. |
SelectedItems | An object collection of the selected items. |
SelectionMode | Specifies the number of items you can select at the same time.
|
ScrollAlwaysVisible | Tells whether the scrollbars are always visible regardless of the number of items in the ListBox. |
Sorted | Tells whether to sort the items in the ListBox alphabetically or in ascending order. |
Text | If you set a string value, the first item that matches will be selected. This property returns the text of the first selected item. |
Figure 1 – ListBox Properties
The following are the useful methods you can use.
Methods | Description |
---|---|
ClearSelected() | Unselects every single chosen thing of the List Box. |
FindString() | Finds the primary item within the ListBox that starts with the required string. The search starts at a such index. |
FindStringExact() | Finds the main thing in the ListBox that matches the predefined string. |
GetSelected() | Tells whether the thing in the predetermined list is chosen. |
SetSelected() | Selects or deselects the item at the specified index. |
Figure 2 – ListBox Methods
To manipulate the items in the ListBox, we use the Items property which is of type ObjectCollection. You can use the typical collection methods such as Add, Remove, and Clear.
Create a new Windows Form and add a ListBox and a TextBox. Set the TextBox‘s Multiline property to true. Follow the layout shown below.
Name the ListBox listBoxInventory and the TextBox textBoxDescription. Double-click the form to add a Load event handler to it. Use the Form1_Load handler(line 16-29) of Example 1.
using System;
using System.Collections.Generic;
using System.Windows.Forms;
namespace ListBoxDemo
{
public partial class Form1 : Form
{
private Dictionary<string, string> products;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
products = new Dictionary<string, string>();
products.Add("Shampoo", "Makes your hair beautiful and shiny.");
products.Add("Soap", "Removes the dirt and germs on your body.");
products.Add("Deodorant", "Prevents body odor.");
products.Add("Toothpaste", "Used to clean your teeth.");
products.Add("Mouthwash", "Fights bad breath.");
foreach (KeyValuePair<string, string> product in products)
{
listBoxInventory.Items.Add(product.Key);
}
}
}
}
Example 1
We created a Dictionary collection that has a string key, and a string value. Inside the Load event handler of the form, we added some products together with their description to this collection. Using a foreach loop, we add each product’s name to the ListBox‘s Items property. Not that each item in the generic Dictionary collection is of type KeyValuePari<TKey, TValue>. When you run the program, you will see that the five products can now be seen inside the ListBox. Note that if the height of the ListBox is insufficient to display all the items, then a vertical scrollbar will be visible to the right of the ListBox.
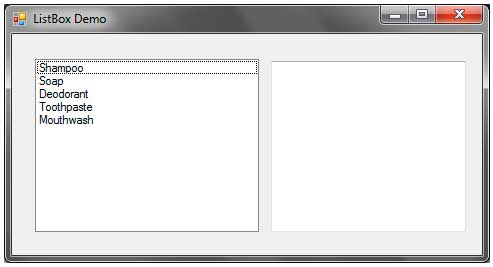
Now let’s add an event handler to the ListBox‘s SelectedIndexChanged event. The SelectedIndexChanged event occurs when the index of the selected item is changed. This is the default event of the ListBox so double clicking the ListBox will automatically add an event handler for the said event. Add this single line of code.
private void listBoxInventory_SelectedIndexChanged(object sender, EventArgs e)
{
textBoxDescription.Text = products[listBoxInventory.Text];
}
Now run the program and select a product. It’s corresponding description should display in the text box.
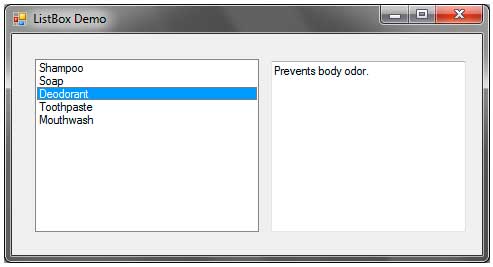
Please note that you can also use the String Collections Editor as shown in the last lesson to add items to the ListBox.