ComboBox Control
The ComboBox control is another way of allowing a user to choose from a set of options. The ComboBox control looks like a text box with a button in its right side. When the button is clicked, the ComboBox show a drop-down box containing the list of options/items available. The user can then choose from these options by clicking one of them. The selected option will then be the text inside the ComboBox. The following are some of the properties of the ComboBox control.
Property | Description |
---|---|
DataSource | A list of data that the control will use to get its items. |
DropDownHeight | The height in pixels of the dropdown box in a combo box. |
FormatString | The format specifier characters that indicate how a value is to be displayed. |
Items | The items in the combo box. |
Sorted | Specifies whether the items on the combo box should be sorted. |
Text | The default text when there is no item selected. |
SelectedIndex | Gets or sets the selected index. Every item has an index starting from 0 to (number of items – 1). A value of -1 indicates that no item is selected. |
SelectedItem | Gets or sets the item of the currently selected item. |
The following table shows some of the events available for the ComboBox control. The default event is the SelectedIndexChanged event.
Event | Description |
---|---|
Click | Occurs when the component is clicked. |
DropDown | Occurs when the drop-down portion of the combo box is shown |
DropDownClosed | Indicates that the drop-down portion of the combo box has been closed. |
SelectedIndexChanged | Occurs when the value of the SelectedIndex the property is changed. |
The following example shows the basic functionality of a ComboBox control. Place a combo box on a blank form. Name it comboBoxNamesby changing the Name property. Go to the Properties Window and find the Items property. You should see a button with three dots in it. Click it to open the String Collection Editor. Alternatively, you can click Edit Items located below the list of properties in the Properties Window.
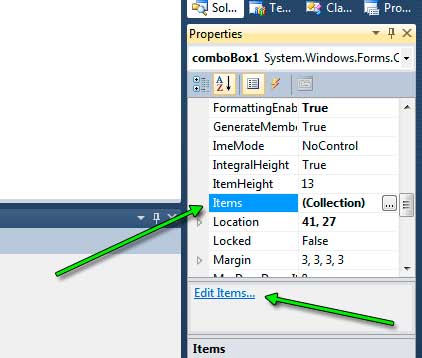
In the String Collection Editor, type the names show in the figure below then press OK.
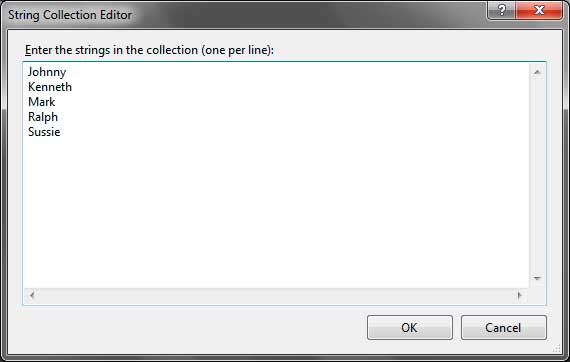
Change the Text property of the combo box to “Choose a name” so it will have a default text when no name is chosen. Double click the combo box so VS/VCE will generate an event handler for the SelectedIndexChanged event. Type the following code inside the event handler.
string selectedName = comboBoxNames.SelectedItem.ToString();
MessageBox.Show("Hello " + selectedName);
Run the program and choose a name. The program will greet the name you have selected immediately after you selected that name.
When you selected an option, the SelectedIndexChanged event is triggered. The SelectedItem property contains the data of the currently selected item. We stored the value of it into a string variable by converting the value of the SelectedItem property into a string using the ToString() method since that property returns an object. (Alternatively you can use the Text property but we used the SelectedItem for demonstration). We then display a text containing the output message.
The SelectedIndex property determines the index of the currently selected item. The first item has an index of 0 and each succeeding items has an index of 1 greater than the previous. The last item has an index of (number of items – 1). So if you have 10 items, the last index is 9. If no item is selected, then the selected index is -1.
If you want to add the items programmatically or during runtime, you can use the Items property of the ComboBox control. The Itemsproperty of type ObjectCollection has an Add method that you can use to add new items to the ComboBox.
string[] names = { "Johnny", "Kenneth", "Mark", "Ralph", "Sussie" };
//Add each names from the array to the combo box
foreach(string name in names)
{
comboBoxNames.Items.Add(name);
}
We created a list of names and stored it in a string array. We then use a foreach loop to add each name to the ComboBox. Alternatively, you can use the DataSource property.
comboBoxNames.DataSource = names;
The DataSource Property can accept a collection or array that the combo box will use to fill its list.